API Documentation
Introduction
The Stagetimer API provides an easy way to remote control your timer with scripts and tools like Bitfocus Companion or vMix.
Most of the endpoints operate on a per-room level, meaning it will interact with the currently highlighted timer. You can see which timer is highlighted by a prominent white border around it on the controller page.
The API is also available for the offline version. You can download the offline version for Mac and Windows from you user dashboard after logging in.
The API is available with any paid license.
How to use this API
If you have never used an API or worked with programming code before, then this page may be confusing to you. API is short for Application Programming Interface, it's a way for two computer programs to talk with each other. For example, you can use this API with the popular Bitfocus Companion software. See this how-to guide for a hands-on example: How to use Stagetimer with Companion for Streamdeck
This documentation uses curl
in the code examples. curl
is nice because you can just copy-paste the code examples into the command line interface of your computer to try them out. On Mac this is the terminal (How to open the terminal), on Windows it's the command prompt (How to open the command prompt).
馃憠 Some API endpoints allow you to supply additional information as --data
. But on Windows you may experience problems with the --data
payload. Alternatively, you can add the them as query parameters, like with the amount
in this example:
https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/tweak?amount=+10m
Authentication
Stagetimer uses simple token-based authentication. An API key is required. You can generate an API key on the controller page.
You can authenticate to the API by providing your API key in the HTTP authorization bearer token header. Alternatively, a slightly lower-security approach is to provide your API key with the api_key
query parameter.
All API requests must be authenticated and made over HTTPS.
# Example using bearer token (recommended)
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/start \
-H "Authorization: Bearer %YOUR_API_KEY%"
# Example using query parameter
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/start?api_key=%YOUR_API_KEY%
Using the index to target timers/messages



Some endpoints operate on a per-timer or per-message level. They require you to define an INDEX
. The INDEX
points to the n-th message in the list on the controller page, starting at 1
. For example, the first timer has INDEX = 1
, the second has INDEX = 2
and so on.
Using the Stagetimer API with the Offline Version
The Stagetimer API is also available for use with the offline version of the software. To use the API with the offline version, you will need to adjust the API endpoint URLs.
For example, instead of using the URL https://api.stagetimer.io/v0/room/YOUR_ROOM_ID/start
as you would with the web version, you will use https://127.0.0.1:3000/api/v0/room/YOUR_ROOM_ID/start
, replacing 127.0.0.1
with the IP address and 3000
with the port number chosen when starting the offline version.
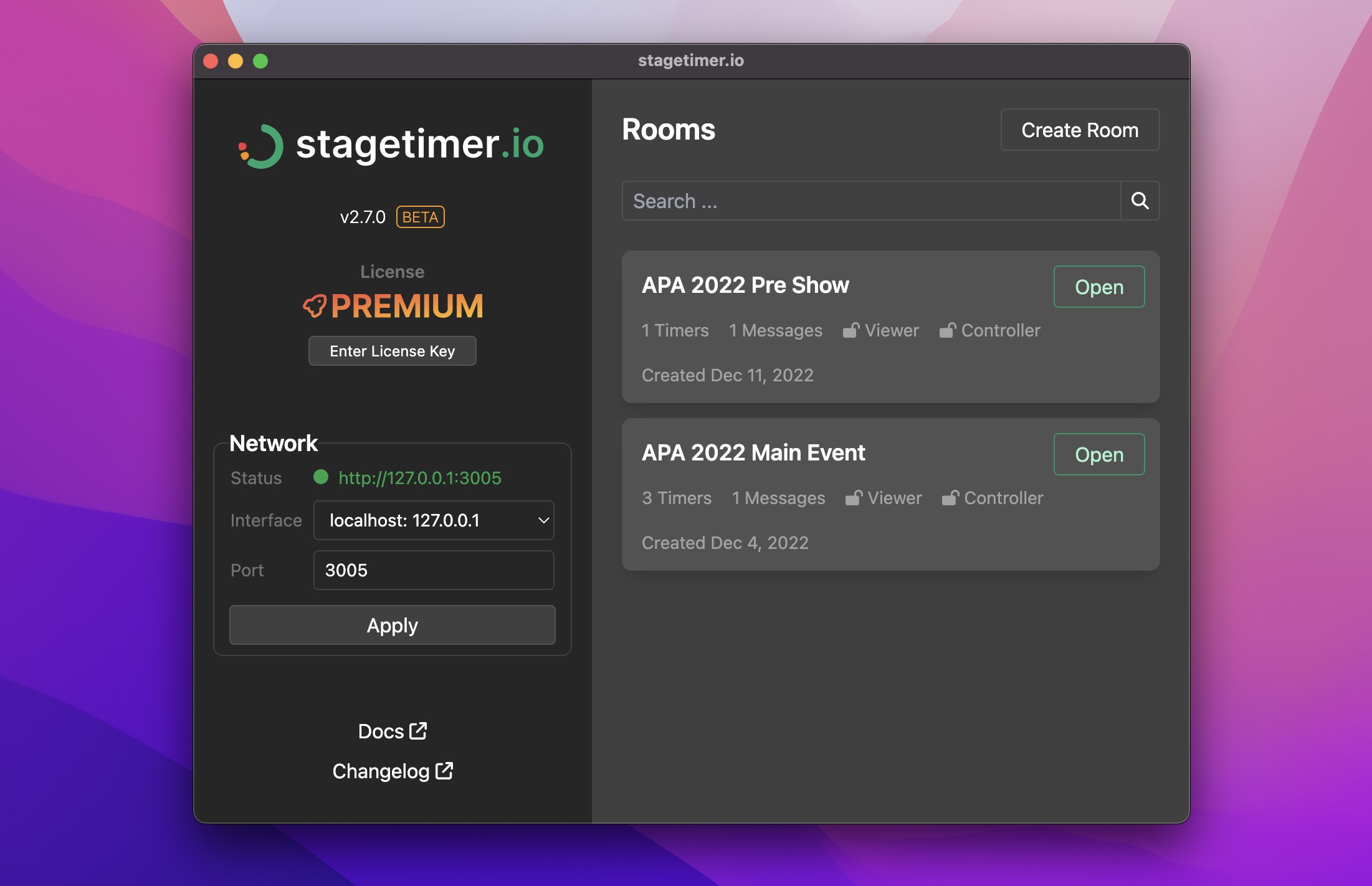
You can download the offline version for Mac and Windows from you user dashboard after logging in. Keep in mind that the API is only available with paid licenses key for the offline version. This includes both the Pro and Premium subscriptions.
Using the API with the offline version allows you to remotely control your timer and automate tasks with scripts and tools like Bitfocus Companion or vMix. Most of the endpoints operate on a per-room level, meaning they will interact with the currently highlighted timer. You can see which timer is highlighted by a prominent prominent blue background on the controller page.
Room endpoints
These endpoints affect the entire room. Most use the currently highlighted timer. A highlighted timer has a blue background when it's paused and a red background when it's running.
POST/room/YOUR_ROOM_ID/start
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/start \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/stop
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/stop \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/toggle
Starts the highlighted timer if it's currently stopped, or stops the timer if it's running. This endpoint is a combination of the start and stop endpoints and toggles between them.
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/toggle \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/reset
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/reset \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/next
Stops the highlighted timer if it's running. Then highlights and sets, if possible, the next timer in the list.
Parameters
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
Name | Description | start optional | 1 start the timer immediately, 0 (default) don't start the timer
|
---|
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/next \
-H "Authorization: Bearer %YOUR_API_KEY%" \
-H "Content-Type: application/json; charset=utf-8" \
--data "{\"start\":0}"
POST/room/YOUR_ROOM_ID/previous
Resets the highlighted timer if it's running. Otherwise activates and sets, if possible, the previous timer in the list.
Parameters
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
Name | Description | start optional | 1 start the timer immediately, 0 (default) don't start the timer
|
---|
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/previous \
-H "Authorization: Bearer %YOUR_API_KEY%" \
-H "Content-Type: application/json; charset=utf-8" \
--data "{\"start\":0}"
POST/room/YOUR_ROOM_ID/tweak
Tweaks the highlighted timer by adding or subtracting time. You have to pass a JSON payload in the body with the amount of time.
Parameters
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
Name | Description | amount required | A string representing the time added or subtracted. For example, +10m adds 10 minutes and -30s subtracts 30 seconds. Valid are any combination of a prefix (+ or - ), a number and a one-letter unit (s for seconds, m for minutes and h for hours). |
---|
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/tweak \
-H "Authorization: Bearer %YOUR_API_KEY%" \
-H "Content-Type: application/json; charset=utf-8" \
--data "{\"amount\":\"+10m\"}"
POST/room/YOUR_ROOM_ID/flash
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/flash \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/blackout
Toggles the blackout on and off.
Parameters
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
Name | Description | force optional | 1 to force enable blackout, 0 to force disable blackout
|
---|
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/blackout \
-H "Authorization: Bearer %YOUR_API_KEY%"
GET/room/YOUR_ROOM_ID/ping
pong
with HTTP status 200 for testing purposes. This enpoint works with GET
, POST
and all other HTTP verbs.
# Try it yourself
curl -v https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/ping \
-H "Authorization: Bearer %YOUR_API_KEY%"
Individual timer endpoints
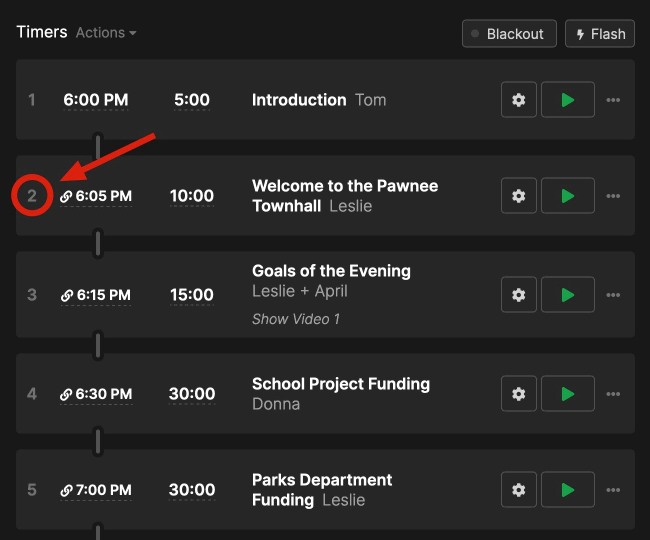
These endpoints affect a specific timer. They require you to define an INDEX
. The INDEX
points to the n-th timer in the list on the controller page, starting at 1
. For example, the first timer has INDEX = 1
, the second has INDEX = 2
and so on.
POST/room/YOUR_ROOM_ID/timer/INDEX/start
INDEX
. If another timer was running, it will stop the previous timer and activate the new timer. If no timer with the given INDEX
exists, then nothing happens.
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/timer/1/start \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/timer/INDEX/stop
INDEX
, but only if it's the currently highlighted one. If no timer with the given INDEX
exists, then nothing happens.
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/timer/1/stop \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/timer/INDEX/toggle
Starts or stops the timer with the given INDEX
. This endpoint is a combination of the start and stop endpoints and toggles between them.
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/timer/1/toggle \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/timer/INDEX/set
Sets the timer with the given INDEX
. If the timer is running, it is reset. If no timer with the given INDEX
exists, then nothing happens.
Parameters
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
You can use these parameters in two ways:
1. In the POST body: --data "{"key":"value"}"
(preferred)
2. As query parameter by adding ?keyA=valueA&keyB=valueB
to the API url
Name | Description | start optional | 1 start the timer immediately, 0 (default) don't start the timer
|
---|
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/timer/1/set \
-H "Authorization: Bearer %YOUR_API_KEY%" \
-H "Content-Type: application/json; charset=utf-8" \
--data "{\"start\":0}"
POST/room/YOUR_ROOM_ID/timer/INDEX/reset
Resets the running timer with the given INDEX
. If no timer with the given INDEX
exists, then nothing happens.
馃憠 Technically identical to /room/YOUR_ROOM_ID/timer/INDEX/set
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/timer/1/reset \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/timer/INDEX/flash
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/timer/1/flash \
-H "Authorization: Bearer %YOUR_API_KEY%"
Individual message endpoints
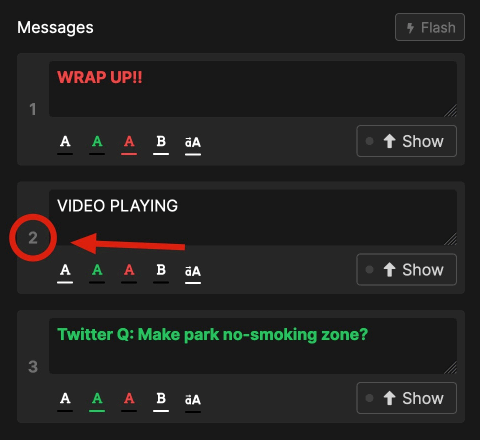
These endpoints affect a specific message. They require you to define an INDEX
. The INDEX
points to the n-th message in the list on the controller page, starting at 1
. For example, the first message has INDEX = 1
, the second has INDEX = 2
and so on.
POST/room/YOUR_ROOM_ID/message/INDEX/show
INDEX
. If no message with the given INDEX
exists, then nothing happens.
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/message/1/show \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/message/INDEX/hide
INDEX
. If no message with the given INDEX
exists, then nothing happens.
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/message/1/hide \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/message/INDEX/toggle
INDEX
. This endpoint is a combination of the show and hide endpoints and toggles between them.
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/message/1/toggle \
-H "Authorization: Bearer %YOUR_API_KEY%"
POST/room/YOUR_ROOM_ID/message/INDEX/flash
# Try it yourself
curl -v -X POST https://api.stagetimer.io/v0/room/%YOUR_ROOM_ID%/message/1/flash \
-H "Authorization: Bearer %YOUR_API_KEY%"
Conclusion
The Stagetimer API allows users to remotely control their timer with scripts and tools such as Bitfocus Companion or vMix. The API uses token-based authentication and offers various endpoints that operate on a per-room, per-timer, or per-message level. Users can start, stop, reset, or tweak timers, as well as change messages and control the room's appearance. The API can be used with the popular Bitfocus Companion software and requires a stable internet connection and an updated browser. A Pro or Premium subscription grants access to additional features and an offline device license.